Building your first page with the Visual Framework 2.0
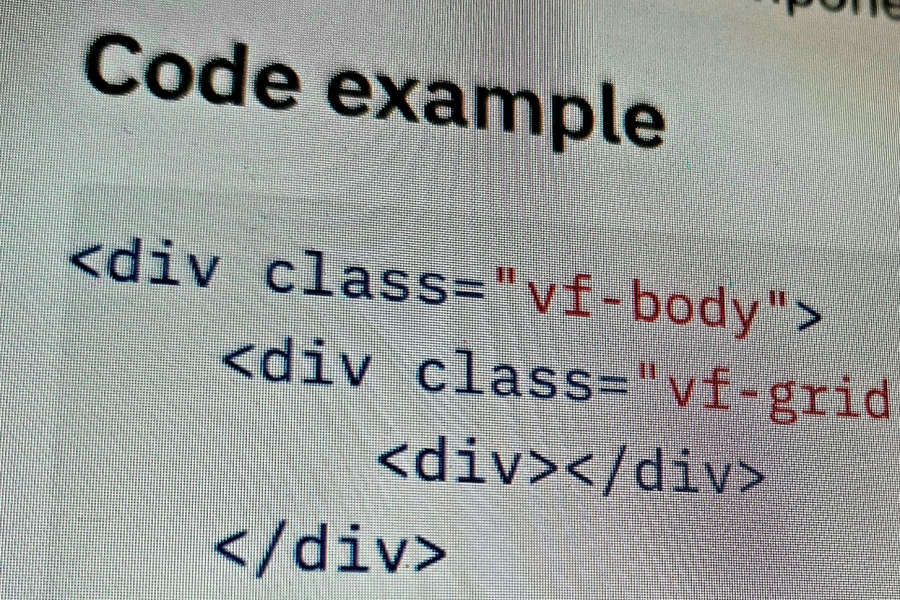
A quick start on how to work with Visual Framework 2.0 CSS, JS and structure your HTML — and lots of links to learn more.
The Visual Framework (VF) is designed to help build life science websites and services, this post is a kick-start to building sites with VF CSS, JS and HTML concepts.
You’ll learn:
- Part 1: Where to find VF documentation
- Part 2: Basic methodologies of VF CSS and JS
- Part 3: How to structure your HTML and CSS classes
- Part 4: Assorted tips and differences from other major frameworks (like Bootstrap)
This post assumes:
- You are familiar with HTML, CSS and JS
- You have probably used Bootstrap or Foundation
- It is helpful to have knowledge of Sass and CSS Custom properties
- You want to use already-built CSS and JS (To build VF components from their Sass and JS modules see this guide)
- You may or may not have used the EMBL-EBI Visual Framework 1.0
Part 1
Where to find VF documentation
To see demonstrations of each component and the HTML and CSS syntax of all “core” Visual Framework components, go to the “VF 2.0 Component library”.
Important: your organisation or project may have added custom components or removed some default components, if so you should ask the maintainers of your design system or component library.
You can always see which components (and which version) are enabled by viewing the CSS source; from the VF demo component library:
/*!
* Component: @visual-framework/vf-badge
* Version: 1.0.0-beta.8
* Location: components/vf-badge
The default VF build will maintain these comments even in the minified CSS build. For higher level information, see the Visual Framework Welcome site.
Part 2
CSS and JS methodologies
To optimise compatibility and flexibility, the Visual Framework does not target HTML elements like <body>
<div>
or <button>
and instead relies only on CSS classes.
Below is an example for a "large heading".
<h2 class="vf-text vf-text-heading--3">This heading size is large</h2> <span class="vf-text vf-text-heading--3">This heading size is large</span> <link rel="stylesheet" href="https://dev.assets.emblstatic.net/vf/v2.0.0-beta.6/css/styles.css">
👉 Tip: This approach is similar to CSS Modules.
The VF is primarily focused on display and structure and therefore has minimal JS. This also helps if you’d like to use, say, Bootstrap for tab behaviour but use the VF look.
Similar to our CSS approach, the VF JS never targets HTML elements or even CSS classes and instead uses JS data attributes; for example a partial look at vf-tabs
:
<div class="vf-tabs-content" data-vf-js-tabs-content>
<section class="vf-tabs__section" id="vf-tabs__section--1">
<h2>Section 1</h2>
The Visual Framework uses class="vf-tabs-content"
for the visual styling and data-vf-js-tabs-content
for the JS — exclusively. Use the VF JS where it’s helpful, if you don’t need it, don’t add the data-*
and use your own JS.
With that in mind, we’ll carry on and focus on the CSS.
Part 3
The structure of HTML, CSS classes
There are a few principles to understand when working with Visual Framework:
- The grid and HTML nesting
The VF makes use of CSS Grid Layout which is quite clever and you should have some basic understanding of how it works, but here are some common tips.
- By default
vf-grid
will try to put your content into columns, if you only want one column:
<div class="vf-grid">
<div>All child content here</div>
</div>
<div class="vf-grid">
<div>This layout</div>
<div>Makes two columns</div>
</div>
<div class="vf-grid"> <div>All child content here</div> </div> <div class="vf-grid"> <div>This layout</div> <div>Makes two columns</div> </div> <link rel="stylesheet" href="https://dev.assets.emblstatic.net/vf/v2.0.0-beta.6/css/styles.css">
.vf-grid > * { border:1px solid; } .vf-grid { margin-bottom: 1rem }
<div class="vf-grid vf-grid__col-3"> <p>1</p> <p>1</p> <p>1</p> <p>1</p> <p>1</p> <p>1</p> </div> <link rel="stylesheet" href="https://dev.assets.emblstatic.net/vf/v2.0.0-beta.6/css/styles.css">
.vf-grid > * { border:1px solid; } .vf-grid { margin-bottom: 1rem }
- If you want to make a custom layout
<div class="vf-grid vf-grid__col-3">
<div>I cover 1 of 3</div>
<div class="vf-grid__col--span-2">I cover 2 of 3 columns</div>
</div>
<div class="vf-grid vf-grid__col-3"> <div>I cover 1 of 3</div> <div class="vf-grid__col--span-2">I cover 2 of 3 columns</div> </div> <link rel="stylesheet" href="https://dev.assets.emblstatic.net/vf/v2.0.0-beta.6/css/styles.css">
.vf-grid > * { border:1px solid; } .vf-grid { margin-bottom: 1rem }
The grid layout often expects child elements. If something doesn't look write, you're probably missing a <div></div>
.
- What dashes and underscores mean
We namespace all universal components. For Visual Framework core components it is a prefix of vf-
. This ensures that the component will not break an existing codebase.
Components in the Visual Framework follow the BEM naming convention:
.vf-component {}
.vf-component__item {}
.vf-component--alternative {}
- Pipes, mixes and chaining CSS classes
You may find that a component contains classes from another. This is a “BEM mix”, it might look like:
<header class="vf-page-header">
<h1 class="vf-page-header__heading | vf-text vf-text--heading-l">Page title</h1>
</header>
Here the vf-page-header
is utilising styling from the vf-text
component.
When mixing classes you’ll see the pipe |
character as a divider, it has no functional difference but improves the readability.
For more, see the guidance on mixes.
- Fake it until you make it
If you’ve structured your component correctly but it doesn’t quite achieve what you need (and you can’t make your own local component) make use of the utility classes; for example:
.vf-u-type__text-body--1
.vf-u-display-none
.vf-u-background-color--red
<div class="vf-button | vf-u-background-color--red">I'm red</div> <link rel="stylesheet" href="https://dev.assets.emblstatic.net/vf/v2.0.0-beta.6/css/styles.css">
- Use
vf-content
for content
Most projects will have larger areas of text where it is either not technically possible or practical to add many classes.
For those situations, wrap the content in a parent element with .vf-content
<section class="vf-content">
<h1>My WYSIWYG-made text</h1>
<p>I'm some user-made content with <a href="#">a link</a>.</p>
</section>
<section class="vf-content"> <h1>My WYSIWYG-made text</h1> <p>I'm some user-made content with <a href="#">a link</a> and I'm not using any element-level VF classes.</p> </section> <link rel="stylesheet" href="https://dev.assets.emblstatic.net/vf/v2.0.0-beta.6/css/styles.css">
Part 4
Assorted tips, differences from other frameworks
A grab-bag of important things that you might not expect.
- Visual Framework defers to your other CSS
If you have a <section class="my-local-style vf-tabs">
the VF CSS is designed to defer to any CSS you have for section {}
and .my-local-style
.
<ul class="nav nav-tabs"> <li class="nav-item"> <a class="nav-link active" href="#">Active</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Bootstrap tabs</a> </li> </ul> <br/> <div classs="vf-tabs"> <ul class="vf-tabs__list"> <li class="vf-tabs__item"> <a class="vf-tabs__link is-active" href="#">Active</a> </li> <li class="vf-tabs__item"> <a class="vf-tabs__link" href="#">VF Tabs</a> </li> </ul> </div> <link href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" rel="stylesheet"> <link rel="stylesheet" href="https://dev.assets.emblstatic.net/vf/v2.0.0-beta.6/css/styles.css">
- Tip: loading the VF CSS after your other CSS can affect this, latter-loaded CSS is favoured by the browser.
- Low specificity
We avoid CSS specificity to target styling of elements. This improves flexibility of HTML structure and allows simpler chaining of classes.
That is:
- We do:
.vf-tabs-content {}
- We don’t:
.vf-tabs .vf-tabs-content {}
- Highly modular
Like most Frameworks, the Visual Framework can be overridden or components can be selectively used. However, the Visual Framework takes this to the next level, allowing selective installation of components and a broad mix of component versions.
For your organisation, the Visual Framework core documentation will likely not be the best source of truth on a component’s HTML, CSS and JS.
So in addition to reading guidance like this, you’ll want to consult the maintainer of your CSS and design systems about which versions of Visual Framework components they’re using and the best place for documentation on your systems.
If you’re lost, you can always as the Slack group for help.
- Atomic Design-like
The Visual Framework makes use of Atomic Design-style concepts to define components. Instead of using Atoms, Molecules, Organisms the Visual Framework uses the terms Elements, Blocks, and Containers.
For more on blocks, elements and containers, see the CSS guidelines
Bonus
Just give me a starter template
📑 Here’s a simple boilerplate so you can see a simple page in action.